Simple bash script for encrypted chat over keybase fs (kbfs)
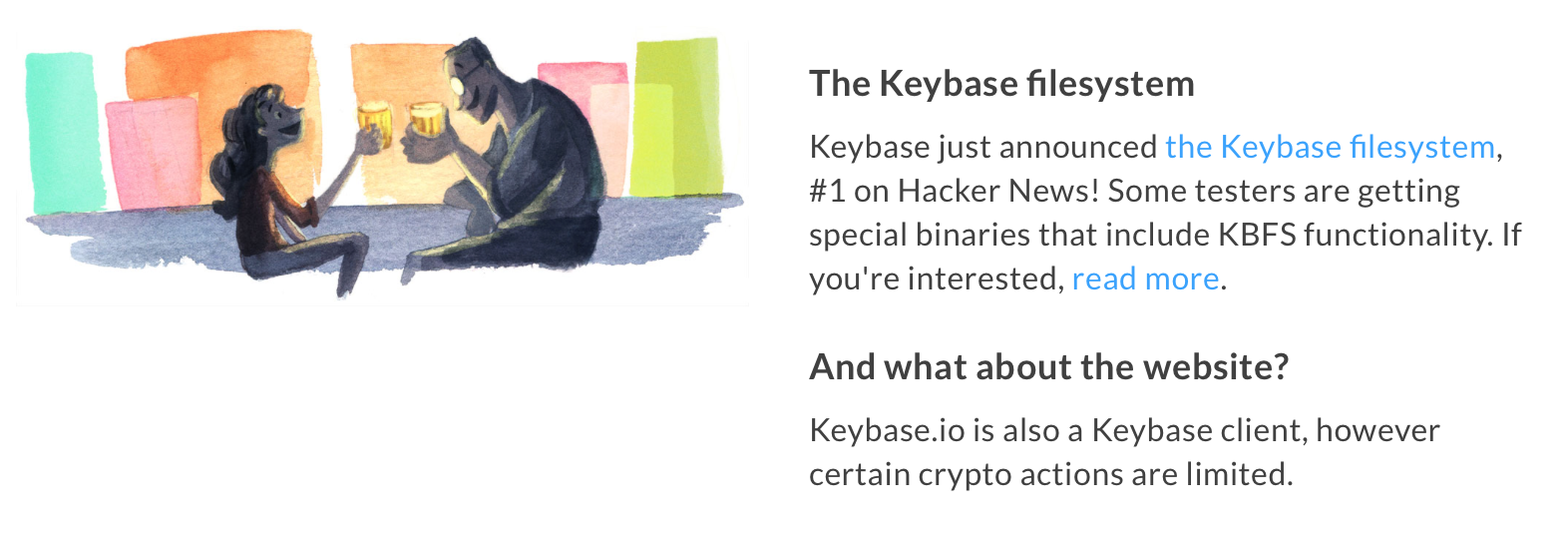
The keybase filesystem is an amazing idea and I was hooked from the first moment I read the keybase.io announcement.
Yesterday, I had the privilege to get access to their alpha, and I have to say that I'm very impressed.
I'm a big fan of filesystem-based "APIs" and kbfs is the kind of infrastructure that makes is it very simple to do complex things.
Yes, kbfs makes it simple to share files with friends (if you didn't follow the link at the beginning of this post, do it now, for some interesting examples) . But, it would also be trivial to implement a secure password managed over kbfs, that keeps passwords synchronised across all your devices.
Or make a secure, encrypted, chat app in just a few lines of bash.
I tried exactly this with my friend @koukopoulos earlier today. We started by using tail -f /keybase/private/vrypan,kouk/chat.log
and `echo Hello >> /keybase/private/vrypan,kouk/chat.log.
Then @kouk turned it into a small bash function, and later I made it into a script called kbtell
.
I can type kbtell kouk -f &
to start following our chat, and kbtell kouk Hey, check my latest blog post.
to send him a message. And our whole chat log is always available at /keybase/private/vrypan,kouk/kbtell.log
.
#! /bin/bash
kbtell_usage() {
echo "Usage: "
echo " kbtell <user> <message>"
echo " kbtell <user> -f"
echo " kbtell <user> -l"
echo
echo " -f to follow (tail) the log, -l to list all messages"
}
[ -z "$kbtell_me" ] && echo "Please set \$kbtell_me to your keybase username" && exit 1
you=$1 ; shift
if [ -z "$you" ] || [ $# -eq 0 ] ; then
kbtell_usage
exit 1
fi
kbtell_log=/keybase/private/$kbtell_me,$you/kbtell.log
if [ ! -f $kbtell_log ] ; then
touch $kbtell_log
fi
if [ $1 = '-f' ]; then
tail -f $kbtell_log
exit 0
elif [ $1 = '-l' ]; then
cat $kbtell_log
exit 0
else
D=`date "+%Y-%m-%d %H:%M:%S"`
msg=$*
out="$D [$kbtell_me] $msg"
echo $out >> $kbtell_log
fi
This is just a quick-n-dirty script. However, it would be very easy to write something in python (or your language of choice) that stores messages in json, and shows the chat in HTML, allows attachments (just put the file in our shared folder and link to it), etc.
I'm really looking forward to kbfs getting out of alpha.